Laravel, a robust PHP framework, and AJAX, a client-side script that communicates to and from a server/database without the need for a page refresh, are pivotal in modern web development. Integrating AJAX in Laravel applications enhances user experience by enabling faster, dynamic, and asynchronous page updates.
This comprehensive guide delves into the essentials of Laravel AJAX, covering everything from basic setup and core concepts to advanced techniques and best practices. We will explore how to effectively implement AJAX in Laravel, tackle common challenges, and answer frequently asked questions, ensuring you have a well-rounded understanding of this powerful combination.
Table of Contents
Understanding Laravel and AJAX
Laravel: A Modern PHP Framework
Laravel, renowned for its elegant syntax, is a contemporary PHP framework that simplifies tasks like routing, authentication, sessions, and caching. It’s designed for developers who need a simple yet powerful toolkit to build full-featured web applications. Laravel’s core features include:
- Eloquent ORM: An advanced implementation of the active record pattern, making database interactions intuitive and efficient.
- Blade Templating Engine: A lightweight yet powerful templating engine, allowing for clean and reusable code.
- MVC Architecture: Ensures a clear separation of business logic and presentation, enhancing maintainability.
- Artisan Console: A built-in tool for performing repetitive and complex tasks effortlessly.
- Security: Robust security features, including protection against SQL injection, cross-site request forgery, and cross-site scripting.
These features, coupled with comprehensive documentation and a supportive community, make Laravel a go-to choice for modern web development.
AJAX: Asynchronous JavaScript and XML
AJAX stands for Asynchronous JavaScript and XML. It’s a set of web development techniques enabling web applications to send and retrieve data from a server asynchronously, without interfering with the display and behavior of the existing page. The process involves:
- Sending a Request: A JavaScript call sends a request to the server using the
XMLHttpRequest
object. - Processing on the Server: The server processes the request, often interacting with a database.
- Response and Update: The server sends a response back, which JavaScript uses to update the web page dynamically.
The benefits of AJAX in web development are significant:
- Improved User Experience: AJAX allows for updating parts of a web page, without reloading the entire page, leading to a smoother, more interactive user experience.
- Reduced Server Load: Since only data is transferred back and forth, the overall load on the server is reduced.
- Asynchronous Operations: Multiple operations can occur in parallel, enhancing the application’s performance.
Integrating AJAX with Laravel brings the best of both worlds: the robust, server-side capabilities of Laravel and the dynamic, user-friendly front-end experience powered by AJAX. This combination is particularly powerful for creating responsive, data-driven web applications.
Setting Up Your Laravel Environment for AJAX
Before diving into the integration of AJAX with Laravel, it’s essential to set up a proper Laravel environment. This setup involves a few key steps, ensuring that your development environment is ready for AJAX implementation.
Laravel Installation Prerequisites
To get started with Laravel, you need to ensure your system meets the following prerequisites:
- PHP: Laravel requires PHP 7.3 or higher. It’s crucial to have the latest PHP version for better performance and security.
- Composer: Composer is a dependency manager for PHP, used for installing Laravel and managing its dependencies.
- Database: Laravel supports various databases like MySQL, PostgreSQL, SQLite, and SQL Server. Ensure you have access to one of these databases.
- Web Server: A web server like Apache or Nginx is required to serve your Laravel application.
Creating a New Laravel Project
Once the prerequisites are in place, you can create a new Laravel project by following these steps:
- Install Laravel: Use Composer to install Laravel globally by running
composer global require laravel/installer
. - Create Project: Create a new project using the Laravel installer with the command
laravel new project-name
, replacingproject-name
with your desired project name. - Environment Configuration: Set up your
.env
file with appropriate database connections and other environment-specific settings.
Configuring Laravel for AJAX Use
To configure Laravel for AJAX, you need to focus on routes and CSRF protection:
- Routes: Define routes in your
web.php
file. AJAX requests in Laravel are handled just like regular requests. You can define routes that respond to AJAX calls specifically by checking for AJAX requests in your controller using therequest()->ajax()
method. - CSRF Protection: Laravel’s
VerifyCsrfToken
middleware, which is applied to your routes by default, provides protection against cross-site request forgery (CSRF) attacks. When making POST requests using AJAX, ensure to include a CSRF token in your request. You can usecsrf_token()
function in your blade template to get the token value.
$.ajax({
url: '/your-route',
type: 'POST',
data: {
_token: '{{ csrf_token() }}',
// Other data
},
success: function(response) {
// Handle success
}
});
With these steps, your Laravel environment is now primed for AJAX development. This setup forms the foundation upon which you can build dynamic, responsive web applications using Laravel and AJAX.
AJAX in Laravel: The Basics
Integrating AJAX into Laravel applications begins with a fundamental understanding of the AJAX request-response cycle and how it interacts with Laravel’s features like Blade templates and routing.
Understanding the Request-Response Cycle
The AJAX request-response cycle in Laravel is a two-way communication process between the client-side and server-side:
- Initiating the Request: An AJAX request is triggered on the client side, typically in response to a user action like clicking a button or submitting a form. This is done using JavaScript or jQuery.
- Processing the Request: The request is sent to a specific route in the Laravel application. Laravel then processes the request, often involving database queries or other logic.
- Sending the Response: Laravel sends back a response, which could be in various formats like JSON, HTML, or plain text.
- Updating the UI: The client-side JavaScript processes the response and updates the user interface accordingly, without needing a page reload.
AJAX and Laravel Blade Templates
Laravel’s Blade templating engine can be effectively used with AJAX. Blade allows you to create dynamic HTML templates, which can include JavaScript code for AJAX requests. Here’s how they interact:
- Embedding JavaScript: You can write JavaScript directly in Blade templates or include external JavaScript files.
- Passing Data: Blade templates can pass data to JavaScript, which can be used in AJAX requests. For example, you can pass a CSRF token or a URL for the AJAX request.
<script>
var url = "{{ route('ajax.route') }}";
var token = "{{ csrf_token() }}";
</script>
Implementing AJAX in Laravel Routes
In Laravel, AJAX requests are handled through routes, just like regular HTTP requests. Here’s how to set it up:
- Define the Route: Create a route in
web.php
orapi.php
that listens for AJAX requests.
Route::post('/ajax-request', 'AjaxController@handleRequest')->name('ajax.route');
- Create a Controller Method: In the corresponding controller, define a method to handle the AJAX request. This method processes the request and returns a response.
public function handleRequest(Request $request) {
// Process request
return response()->json(['success' => 'Response from AJAX']);
}
- JavaScript AJAX Call: On the client side, use JavaScript or jQuery to make the AJAX call to the defined route.
$.ajax({
type: "POST",
url: url,
data: { _token: token },
success: function(response) {
console.log(response.success);
}
});
Understanding these basics of AJAX in Laravel sets the stage for creating interactive and dynamic web applications. By leveraging Laravel’s routing and Blade templates, developers can efficiently handle AJAX requests and create seamless user experiences.
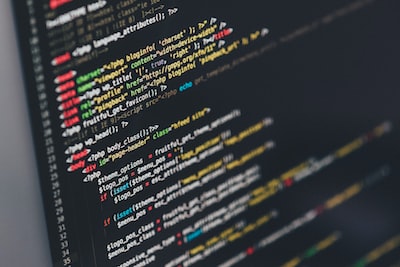
Advanced AJAX Techniques in Laravel
As you become more comfortable with AJAX in Laravel, you can explore advanced techniques that enhance functionality and user experience. These include sophisticated use of controllers, handling JSON responses effectively, and implementing robust error handling and validation in AJAX requests.
Using Laravel Controllers with AJAX
Laravel controllers play a crucial role in managing the logic of AJAX requests. Here’s how to leverage them effectively:
- Complex Logic Handling: Controllers can handle complex business logic before sending a response. This includes interacting with models, database queries, and other application-specific logic.
- Resource Controllers: For CRUD operations, Laravel’s resource controllers provide a convenient way to handle AJAX requests. Each resource route corresponds to a controller method.
- Response Methods: Laravel provides various response methods suitable for AJAX, like
response()->json()
for JSON responses.
public function update(Request $request, $id) {
$item = Item::findOrFail($id);
// Update logic
return response()->json(['message' => 'Item updated successfully']);
}
Handling JSON Responses
JSON is the preferred format for AJAX responses in Laravel due to its lightweight and easy-to-parse nature.
- Creating JSON Responses: Use Laravel’s
response()->json()
method to send JSON responses. This method automatically sets the correct content type header. - Data Transformation: You can transform your data using Laravel’s Eloquent resources or collections before converting it to JSON, ensuring the response is in the desired format.
return response()->json(ItemResource::collection(Item::all()));
Error Handling and Validation in AJAX Requests
Robust error handling and validation are essential for a seamless user experience.
- Validation: Laravel’s validation features can be used in AJAX requests. Use the
validate()
method in your controller to validate incoming data.
$request->validate([
'name' => 'required|max:255',
// Other validation rules
]);
- Error Responses: In case of validation errors, Laravel automatically generates a JSON response with error details, which can be displayed on the client side.
- Custom Error Handling: You can also create custom error responses using try-catch blocks or exception handling to manage unexpected server-side errors.
try {
// Process request
} catch (\Exception $e) {
return response()->json(['error' => $e->getMessage()], 500);
}
By mastering these advanced AJAX techniques in Laravel, you can create more efficient, reliable, and user-friendly web applications. These practices ensure that your application not only functions well but also provides a robust and engaging user experience.
Real-World Examples of AJAX in Laravel
Implementing AJAX in Laravel can significantly enhance the functionality and user experience of web applications. Let’s explore some real-world examples where AJAX can be effectively used in Laravel projects.
Example 1: AJAX-based Form Submission
One common use of AJAX in Laravel is for form submissions. This allows for submitting forms without refreshing the page, providing a seamless user experience.
- Frontend: A typical form in a Blade template, with a JavaScript function to handle the form submission using AJAX.
<form id="myForm">
<input type="text" name="name">
<button type="submit">Submit</button>
</form>
<script>
$('#myForm').on('submit', function(e) {
e.preventDefault();
$.ajax({
type: 'POST',
url: '/submit-form',
data: $(this).serialize(),
success: function(response) {
alert('Form submitted successfully');
}
});
});
</script>
- Backend: In the Laravel controller, handle the form submission and return a JSON response.
public function submitForm(Request $request) {
// Form processing logic
return response()->json(['message' => 'Form submitted successfully']);
}
Example 2: Dynamic Data Loading with AJAX
AJAX can be used to dynamically load data into a page, such as loading more content when a user scrolls to the bottom of a page.
- JavaScript Function: A function that triggers when the user scrolls to the bottom, sending an AJAX request to load more content.
$(window).scroll(function() {
if($(window).scrollTop() == $(document).height() - $(window).height()) {
// AJAX call to get more content
}
});
- Laravel Backend: A controller method that fetches the next set of data and returns it as a JSON response.
public function loadMoreData(Request $request) {
$data = DataModel::paginate(10);
return response()->json($data);
}
Example 3: Real-time Search Functionality
Implementing real-time search functionality using AJAX allows users to get search results as they type, without reloading the page.
- Search Input: A search box in a Blade template with an on-key-up event to trigger the AJAX call.
<input type="text" id="search" onkeyup="searchFunction()">
- AJAX Call: A JavaScript function that sends the search query to the server.
function searchFunction() {
var query = $('#search').val();
$.ajax({
type: 'GET',
url: '/search',
data: { 'search': query },
success: function(data) {
// Update the page with search results
}
});
}
- Laravel Route and Controller: A route that handles the search request and a controller method that returns search results.
public function search(Request $request) {
$results = SearchModel::where('name', 'like', '%' . $request->search . '%')->get();
return response()->json($results);
}
These examples demonstrate the versatility and efficiency of using AJAX in Laravel applications, enabling dynamic content loading, real-time interactions, and improved user experiences without the need for full page reloads.
Best Practices for Laravel AJAX Development
When developing with Laravel and AJAX, adhering to best practices not only enhances code quality but also ensures a secure and efficient application.
Code Organization and Structure
- Modularization: Keep AJAX-related JavaScript code separate from Blade templates. Use external JavaScript files or dedicated script sections in your Blade files.
- Use of Controller Methods: Handle AJAX requests in specific controller methods. This keeps your logic organized and makes your code easier to maintain.
- Route Naming: Clearly name your routes for AJAX requests. This makes it easier to understand and manage the routes in your application.
Security Considerations
- CSRF Protection: Always include CSRF tokens in your AJAX requests to protect against cross-site request forgery attacks.
- Input Validation: Validate all user inputs on the server side, even if they are validated on the client side, to prevent malicious data from being processed.
- Sanitizing Data: Sanitize data both when receiving inputs and displaying outputs to prevent cross-site scripting (XSS) attacks.
Performance Optimization
- Minimize Data Transfer: Only send necessary data in AJAX requests and responses to reduce load times and server bandwidth usage.
- Caching Strategies: Implement caching for responses that don’t change often, reducing the number of requests to the server.
- Asynchronous Loading: Load data asynchronously and in smaller chunks to improve user experience and reduce server load.
Common Challenges and Solutions
Debugging AJAX Requests in Laravel
- Use Browser Developer Tools: Modern browsers have developer tools that allow you to inspect AJAX requests and responses. This can be invaluable for debugging.
- Logging: Implement logging in your Laravel application to capture details of AJAX requests and potential errors.
Handling Session Timeouts
- Session Timeout Alerts: Implement JavaScript alerts or notifications to inform users when their session is about to expire.
- Automatic Session Extension: Use AJAX to periodically send requests to the server to keep the session alive.
Cross-Origin Resource Sharing (CORS) Issues
- CORS Middleware: Implement CORS middleware in Laravel to handle cross-origin requests. This middleware can be configured to allow specific domains and set headers required for CORS.
- Handling Preflight Requests: Ensure that your Laravel application correctly handles preflight OPTIONS requests that are part of the CORS protocol.
By following these best practices and addressing common challenges, you can create robust, efficient, and secure Laravel AJAX applications that offer a seamless user experience.
FAQs
How to ensure security in Laravel AJAX calls?
Security in Laravel AJAX calls can be ensured by:
Including CSRF tokens in AJAX requests.
Validating and sanitizing user input on the server side.
Implementing proper authentication and authorization mechanisms.
Using middleware to protect routes from unauthorized access.
What are the best practices for error handling in Laravel AJAX?
Best practices for error handling in Laravel AJAX include:
Using try-catch blocks to catch and handle exceptions.
Returning informative error messages and HTTP status codes in JSON responses.
Implementing global error handling using Laravel’s exception handling features.
Logging errors to facilitate debugging and monitoring.
Can AJAX be used with Laravel APIs?
Yes, AJAX can be used with Laravel APIs. You can create API endpoints in Laravel and make AJAX requests to these endpoints to interact with your application’s data. It’s a common practice for creating dynamic and interactive single-page applications (SPAs) that communicate with a Laravel backend through AJAX.
How to optimize AJAX requests for better performance in Laravel?
To optimize AJAX requests in Laravel for better performance:
Minimize the amount of data transferred in AJAX responses.
Implement client-side caching where appropriate.
Use Laravel’s built-in caching mechanisms for frequently used data.
Load data asynchronously and consider lazy loading for non-essential content.
Conclusion
In conclusion, mastering Laravel AJAX is essential for building modern, responsive web applications. AJAX empowers developers to create dynamic and interactive user experiences while leveraging the robust features of the Laravel framework. As you explore the world of AJAX in Laravel, remember to prioritize security, adhere to best practices, and optimize for performance.
We encourage you to experiment with AJAX in your Laravel projects, as it opens up a world of possibilities for creating engaging web applications. For further resources and in-depth guidance on Laravel and AJAX development, refer to Laravel’s official documentation and online communities. Happy coding!