Exim is a mail transfer agent (MTA), which is the software responsible for receiving, routing, and delivering email messages. It’s widely used on Unix-like operating systems and is the default MTA on some Linux distributions. Exim is known for its flexibility, as it allows administrators to control its behavior through a variety of configuration options.
The exim_mainlog
file is Exim’s primary log file. It contains detailed information about the operation of the Exim MTA, including every message that is received, routed, and delivered. Specific data logged includes the message ID, sender, and recipient addresses, the time when the message was received and when it was delivered, and whether the delivery was successful or not. If delivery fails, the log will include an error message that indicates why the delivery failed. This information is invaluable when troubleshooting issues with email delivery. In general, understanding and analyzing exim_mainlog
can help ensure the smooth operation of an Exim-based mail system.
Here’s a basic PHP script that you can use as a starting point:
<?php
// Specify the location of your Exim log
$logFile = '/var/log/exim_mainlog';
// Check if the file exists
if (!file_exists($logFile)) {
die("File not found");
}
// Read the file
$logContent = file_get_contents($logFile);
// Split the content into lines
$lines = explode("\n", $logContent);
// Array to hold the bounced email addresses
$bouncedEmails = [];
foreach ($lines as $line) {
// Check if the line contains a bounce message
if (strpos($line, '**') !== false) {
// Use a regex to extract the email address
preg_match('/[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+/', $line, $matches);
// Add the email address to the array
if (count($matches) > 0) {
$bouncedEmails[] = $matches[0];
}
}
}
// Remove duplicates
$bouncedEmails = array_unique($bouncedEmails);
// Print out the bounced email addresses
foreach ($bouncedEmails as $email) {
echo $email . "\n";
}
?>
This script reads the Exim log file, splits it into lines, and checks each line for a bounce message. If it finds a bounce message, it uses a regular expression to extract the email address and adds it to an array. Finally, it removes any duplicates and prints out the bounced email addresses.
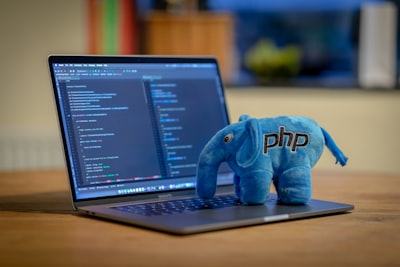
Why is it important to collect failed email addresses?
Blacklisting bounced email addresses is crucial for maintaining the reputation of your sending IP and your email deliverability rate. Continually sending messages to invalid or non-existent addresses, which result in bounces, can lead to being flagged as spam by Internet Service Providers (ISPs). This can cause legitimate emails to also end up in recipients’ spam folders or be blocked entirely. Additionally, it wastes resources as you’re sending emails that will never be received. By promptly identifying and blacklisting bounced email addresses, you can focus on reaching genuine, active recipients, thus improving the efficiency and effectiveness of your email campaigns.
More information about Mail Server Reputation
Email reputation, also known as sender reputation, is a score assigned to a sender’s IP address or domain by Internet Service Providers (ISPs) to determine the legitimacy of outgoing emails. It’s influenced by several factors. High bounce rates, spam complaints, sending to spam traps or blacklisted addresses, and email volume and frequency can negatively affect the reputation. Conversely, consistent sending volumes, low bounce rates, low complaint rates, and good list hygiene practices improve it. High email reputation ensures better deliverability, as ISPs are more likely to direct the emails to the inbox rather than the spam folder. It’s a critical aspect of successful email marketing.