In this blog post, we will guide you through the process of running a Laravel project on Amazon Web Services (AWS) Elastic Kubernetes Service (EKS).
AWS Elastic Kubernetes Service (EKS) provides a range of benefits over self-managed Kubernetes, making it an attractive choice for businesses. EKS eliminates the complexity of setting up, operating, and maintaining Kubernetes clusters, allowing teams to focus on developing applications. It also offers robust scalability and high availability with multi-AZ deployments, ensuring seamless performance during demand spikes. With EKS, you get deep integration with AWS services like RDS, S3, and IAM, enhancing security and efficiency. Additionally, EKS automatically manages updates and patches, reducing the administrative burden and risk of vulnerabilities, and ensuring your applications are always running on the latest, most secure version of Kubernetes.
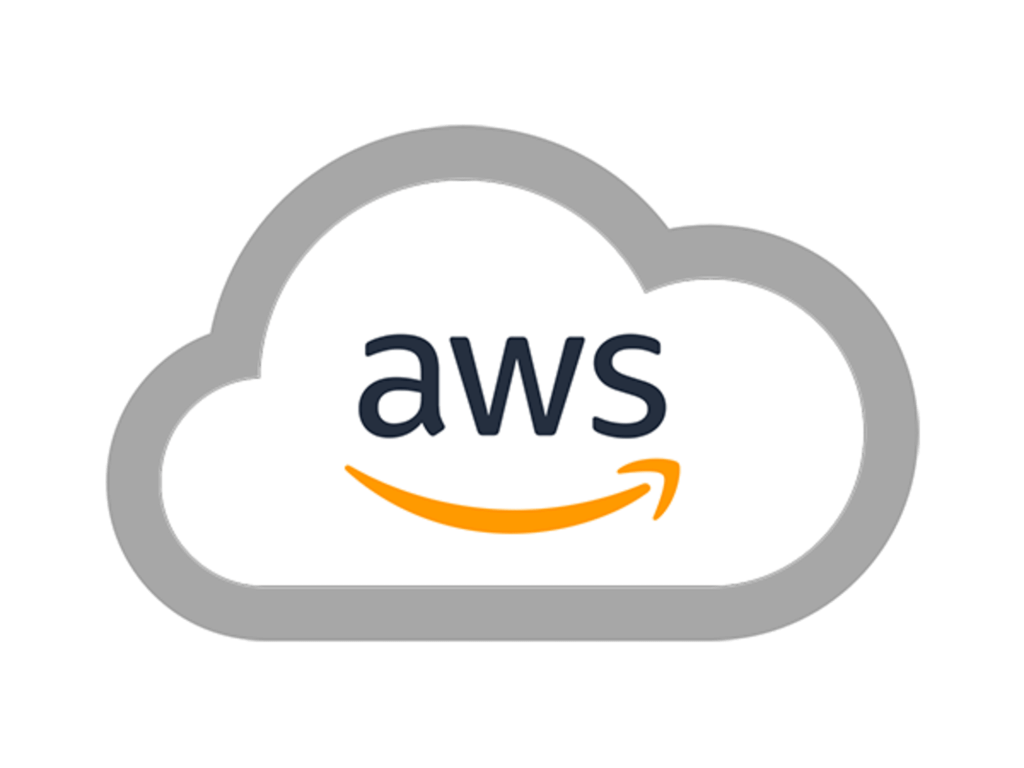
Table of Contents
Prerequisites
Before we get started, here are the prerequisites:
- Basic understanding of AWS services.
- Basic understanding of Laravel, PHP’s framework.
- Basic understanding of Docker and Kubernetes.
- AWS Account with necessary permissions.
- AWS CLI and kubectl installed on your system.
- Docker installed on your system.
- An existing Laravel project.
Step 1: Dockerize Your Laravel Project
The first step is to create a Dockerfile at the root of your Laravel project. The Dockerfile specifies the Docker image and includes all the necessary dependencies to run your Laravel application. Below is a simple example:
FROM php:7.4-fpm
# Install dependencies
RUN apt-get update && apt-get install -y \
build-essential \
libpng-dev \
libjpeg62-turbo-dev \
libfreetype6-dev \
locales \
zip \
jpegoptim optipng pngquant gifsicle \
vim \
unzip \
git \
curl
# Clear cache
RUN apt-get clean && rm -rf /var/lib/apt/lists/*
# Install extensions
RUN docker-php-ext-install pdo_mysql mbstring zip exif pcntl
RUN docker-php-ext-configure gd --with-freetype=/usr/include/ --with-jpeg=/usr/include/
RUN docker-php-ext-install gd
# Install composer
COPY --from=composer:latest /usr/bin/composer /usr/bin/composer
# Set working directory
WORKDIR /var/www
COPY . /var/www
# Install dependencies
RUN composer install
CMD php artisan serve --host=0.0.0.0 --port=8080
EXPOSE 8080
Step 2: Build and Push the Docker Image to AWS ECR
Next, we need to build a Docker image from the Dockerfile and push it to AWS Elastic Container Registry (ECR).
First, create a repository on ECR:
aws ecr create-repository --repository-name laravel-app --region <region>
Build the Docker image:
docker build -t laravel-app .
Authenticate Docker to the Amazon ECR:
aws ecr get-login-password --region <region> | docker login --username AWS --password-stdin <account-id>.dkr.ecr.<region>.amazonaws.com
Tag the Docker image:
docker tag laravel-app:latest <account-id>.dkr.ecr.<region>.amazonaws.com/laravel-app:latest
Push the Docker image:
docker push <account-id>.dkr.ecr.<region>.amazonaws.com/laravel-app:latest
Step 3: Set Up AWS EKS
Now, we will create a Kubernetes cluster using AWS EKS. For this, you need to create a VPC (or you can use an existing one), and then create an EKS cluster.
First, set up the VPC, subnets, and security groups with the necessary IAM roles. You can use AWS Management Console, AWS CLI, or CloudFormation for this.
Next, use the AWS Management Console to create an EKS cluster:
- Open the Amazon EKS console at https://console.aws.amazon.com/eks/.
- Choose Create cluster.
- For Name, enter a unique name for your cluster.
- For Kubernetes version, choose the version you want to use.
- For Cluster service role, choose the IAM role that allows the Amazon EKS service to manage other AWS services on your behalf.
- For VPC, select the VPC you created for this cluster.
- Choose Create.
After creating the cluster, you’ll need to set up the kubectl
command-line tool with the necessary permissions. This can be done by updating your kubeconfig file with the information about your cluster:
aws eks --region <region> update-kubeconfig --name <cluster_name>
Step 4: Create a Deployment and Service in EKS
After setting up the EKS cluster, we will create a deployment that runs our Docker container. Create a deployment.yaml
file:
apiVersion: apps/v1
kind: Deployment
metadata:
name: laravel-deployment
spec:
replicas: 3
selector:
matchLabels:
app: laravel-app
template:
metadata:
labels:
app: laravel-app
spec:
containers:
- name: laravel-app
image: <account-id>.dkr.ecr.<region>.amazonaws.com/laravel-app:latest
ports:
- containerPort: 8080
This deployment creates three replicas of our Docker container. Now, apply the deployment:
kubectl apply -f deployment.yaml
Next, create a service to expose our application to the internet. Create a service.yaml
file:
apiVersion: v1
kind: Service
metadata:
name: laravel-service
spec:
type: LoadBalancer
ports:
- port: 80
targetPort: 8080
selector:
app: laravel-app
This service creates a LoadBalancer that listens to HTTP traffic (port 80) and forwards it to our application (port 8080). Now, apply the service:
kubectl apply -f service.yaml
Step 5: Access Your Laravel Application
After creating the service, it might take a few minutes for AWS to set up the LoadBalancer. Once done, you can get the URL of your application with:
kubectl get service laravel-service
Look for the “EXTERNAL-IP” in the output. This is the URL of your Laravel application.
Now you can access your Laravel application from any web browser using the provided URL.
Congratulations! You’ve successfully deployed your Laravel application on AWS EKS! Level-up your application by implenting a Continuous Integration pipeline in Jenkins.
Conclusion
In this blog post, we’ve covered the steps to Dockerize a Laravel application, create a Docker image, push it to AWS ECR, set up AWS EKS, create a Kubernetes deployment and service, and finally access the Laravel application. AWS EKS offers a scalable and robust environment for deploying Laravel applications, and it’s definitely worth considering for your production workloads.