Restful APIs have become a fundamental building block of modern web applications. They are used to enable communication between different components of an application or different applications themselves. When it comes to developing Restful APIs, two popular web frameworks are Django and Laravel.
Django is a Python-based web framework that provides a rich set of tools and libraries for building web applications. It was originally developed by the Lawrence Journal-World newspaper in 2003 and was released as open source in 2005.
Laravel, on the other hand, is a PHP-based web framework that was developed by Taylor Otwell in 2011. It is built on top of several Symfony components and provides an expressive, elegant syntax for building web applications.
In this blog post, we will compare Django and Laravel for Restful API development based on various factors.
Criteria | Laravel | Django |
---|---|---|
Language | PHP | Python |
Learning Curve | Moderate; easy for those familiar with PHP | Easy to moderate; easy for Python users |
Database support | MySQL, PostgreSQL, SQLite, SQL Server | MySQL, PostgreSQL, SQLite, Oracle |
ORM (Object-relational mapping) | Eloquent ORM | Django ORM |
Routing | Simple and intuitive routing | URL Dispatcher is easy to use |
Template engine | Blade | Django’s own Template Language (DTL) |
Middleware support | Yes, has middleware for HTTP routing | Yes, has middleware for request/response processing |
Security | CSRF protection, SQL injection protection, XSS protection | CSRF and XSS protection, SQL injection protection |
Scalability | Scalable, but may require additional configuration | Highly scalable out of the box |
RESTful Support | Built-in support | Needs Django REST framework add-on |
Community Support | Large and active | Very large and active |
Testing Tools | Built-in testing tools with PHPUnit | Built-in testing tools |
Performance | High performance with proper optimization | High performance with proper optimization |
Documentation | Comprehensive and well-documented | Comprehensive and well-documented |
Authentication System | Built-in, with Laravel Sanctum for API authentication | Built-in, with Django REST Framework’s Token-based authentication |
Real-time capability | Laravel Echo for real-time events | Django Channels for real-time events |
Package manager | Composer | Pip |
Table of Contents
Ease of setup and configuration
Django provides a comprehensive set of tools for setting up and configuring a Restful API. It comes with a built-in ORM (Object Relational Mapper) that makes it easy to work with databases. Django Rest Framework, an open-source toolkit, provides several additional features like authentication, serialization, and content negotiation, making it easy to build Restful APIs.
Laravel also provides a straightforward way to set up and configure a Restful API. It comes with an Eloquent ORM, which is a simple and elegant way to work with databases. Laravel Passport, an open-source package, provides authentication features like OAuth2 and API token authentication, making it easy to secure Restful APIs.
Both frameworks provide an easy-to-use command-line interface (CLI) for generating boilerplate code and scaffolding, making it easy to get started with Restful API development.
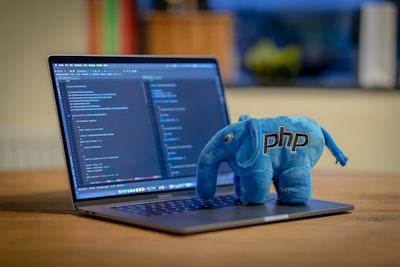
Performance and Scalability
Django is known for its performance and scalability. It has a robust caching system that can cache database queries and templates, improving the speed of the application. Django also supports multiple databases and has built-in support for load balancing, making it easy to scale applications horizontally.
Laravel is also known for its performance and scalability. It has a built-in caching system that can cache database queries, routes, and configurations. Laravel also supports multiple databases and has built-in support for load balancing, making it easy to scale applications horizontally.
Community and Documentation
Django has a vibrant community of developers, with over 15,000 packages available on PyPI (Python Package Index). It has comprehensive documentation that covers all aspects of web development, including Restful API development. Django also has a large number of third-party packages available, providing additional functionality and features.
Laravel also has a large and active community of developers, with over 13,000 packages available on Packagist (PHP Package Repository). It has comprehensive documentation that covers all aspects of web development, including Restful API development. Laravel also has a large number of third-party packages available, providing additional functionality and features.
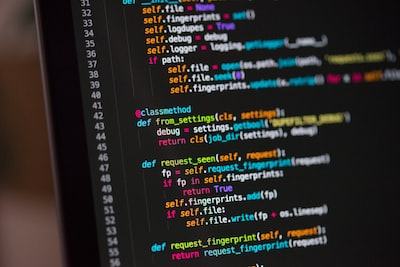
Testing
Both Django and Laravel provide built-in testing frameworks for unit testing, functional testing, and integration testing. Django’s testing framework provides a TestClient that can simulate HTTP requests and responses, making it easy to test Restful APIs. Laravel’s testing framework provides a TestResponse that can simulate HTTP requests and responses, making it easy to test Restful APIs.
Security
Django provides several built-in security features, including protection against SQL injection and cross-site scripting (XSS) attacks. Django also provides built-in support for HTTPS and secure cookies, making it easy to secure Restful APIs.
Laravel also provides several built-in security features, including protection against SQL injection and cross-site scripting (XSS) attacks. Laravel also provides built-in support for HTTPS and secure cookies, making it easy to secure Restful APIs.
Code Examples
Django
# requirements.txt
# Django==3.2.3
# djangorestframework==3.12.4
# myproject/views.py
from rest_framework.views import APIView
from rest_framework.response import Response
class HelloWorld(APIView):
def get(self, request):
return Response({"message": "Hello, world!"})
# myproject/urls.py
from django.urls import path
from .views import HelloWorld
urlpatterns = [
path('hello/', HelloWorld.as_view(), name='hello-world'),
]
# myproject/settings.py
INSTALLED_APPS = [
...
'rest_framework',
]
# myproject/manage.py
# runserver command
Laravel
# requirements.txt
# Django==3.2.3
# djangorestframework==3.12.4
# myproject/views.py
from rest_framework.views import APIView
from rest_framework.response import Response
class HelloWorld(APIView):
def get(self, request):
return Response({"message": "Hello, world!"})
# myproject/urls.py
from django.urls import path
from .views import HelloWorld
urlpatterns = [
path('hello/', HelloWorld.as_view(), name='hello-world'),
]
# myproject/settings.py
INSTALLED_APPS = [
...
'rest_framework',
]
# myproject/manage.py
# runserver command
I hope this comparison will start you off on the right track, let me know what you think of this article by commenting below!