TestNG, a renowned Java testing framework inspired by JUnit, has introduced several functionalities that make it more powerful and flexible, especially for integration tests. Among its myriad features, TestNG DataProviders stand out as a pivotal tool for testers and developers alike.
DataProviders in TestNG are specifically designed to facilitate data-driven testing, a methodology where test cases are executed with multiple sets of data. By employing DataProviders, one can effortlessly pass varied parameters directly to test methods, eliminating the redundancy of writing separate methods for each data set. This not only streamlines the testing process but also enhances the comprehensiveness of tests. The ability to feed diverse data sets into a single test method ensures that the application under test is robust and can handle different input scenarios.
As the demand for efficient and comprehensive testing grows, understanding and leveraging DataProviders becomes indispensable for quality assurance professionals.
Table of Contents
Understanding the Basics of DataProviders
Data-driven testing is like the heartbeat of modern software testing. It ensures that our applications are robust and can handle varied inputs. And when we talk about data-driven testing in TestNG, DataProviders are the unsung heroes.
- Defining DataProviders in TestNG: A DataProvider is essentially a mechanism that allows you to pass different sets of data to your test methods. Imagine having a magic box; every time you open it, you find a different gift (data set) tailored for your test cases.
@DataProvider(name = "testData")
public Object[][] provideData() {
return new Object[][] { {"data1"}, {"data2"}, {"data3"} };
}
- The Great Divide: DataProviders vs. TestNG Parameters: While both DataProviders and TestNG parameters allow you to pass data to your test methods, they have distinct differences. TestNG parameters are like a direct flight – you know your destination and you get there directly. DataProviders, however, are more like a tour where you visit multiple destinations (test values) in one journey.
- Structuring Your DataProviders: The beauty of DataProviders lies in its structure. It’s designed to return a 2D list of objects, allowing you to pass multiple parameters to your test methods. The syntax is straightforward, and once set up, it’s like having a conveyor belt delivering different sets of data right to your test methods.
@DataProvider(name = "loginData")
public Object[][] provideLoginData() {
return new Object[][] { {"user1", "pass1"}, {"user2", "pass2"} };
}
In this code snippet, we’re setting up a DataProvider that provides different sets of usernames and passwords for a login test. It’s efficient, clean, and ensures our tests cover multiple scenarios.
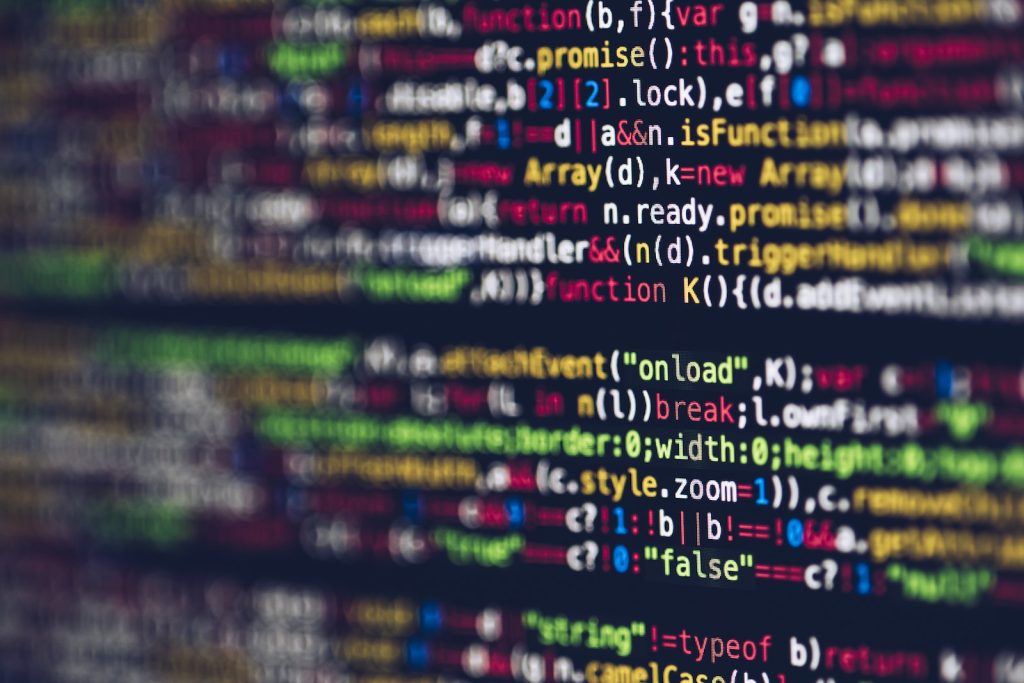
Crafting TestNG DataProviders Like a Pro
In the world of TestNG, the @DataProvider
annotation is akin to a master key, unlocking the doors to dynamic and versatile testing. But like any tool, its true power is harnessed only when you know how to wield it correctly. Let’s dive deep into the syntax and nuances of this mighty annotation.
- Decoding the
@DataProvider
annotation: At its heart, the@DataProvider
annotation in TestNG is a beacon, signaling the framework to fetch data from a specific method. It’s like telling your assistant, “Hey, get the data from that shelf!” But instead of a shelf, it’s a method.
@DataProvider(name = "sampleData")
public Object[][] fetchSampleData() {
return new Object[][] { {"dataA"}, {"dataB"} };
}
In this snippet, the @DataProvider
annotation is used to mark the fetchSampleData
method as the source of test data.
- Declaring and Dancing with DataProviders: Declaring a DataProvider is a breeze. Once you’ve annotated a method with
@DataProvider
, you can use it in your test methods by referencing its name. It’s like having a playlist, and each song (data set) plays when its turn comes up.
@Test(dataProvider = "sampleData")
public void sampleTestMethod(String data) {
System.out.println("Testing with: " + data);
}
Here, the sampleTestMethod
will run twice, once with “dataA” and then with “dataB”, thanks to our dataprovider.
- The Name Game: Why Naming Your DataProvider Matters: In the world of coding, naming is crucial. It’s how we identify and reference things. With DataProviders, naming serves a dual purpose:
- Identification: A unique name helps in quickly identifying the source of data.
- Reference: When linking a test method to a DataProvider, the name acts as the bridge.
But here’s a fun twist! If you decide to play the mysterious card and skip naming your DataProvider, TestNG’s got your back. It will simply use the method’s name as the default DataProvider name. So, while naming is essential, TestNG ensures you have the flexibility to go with the flow.
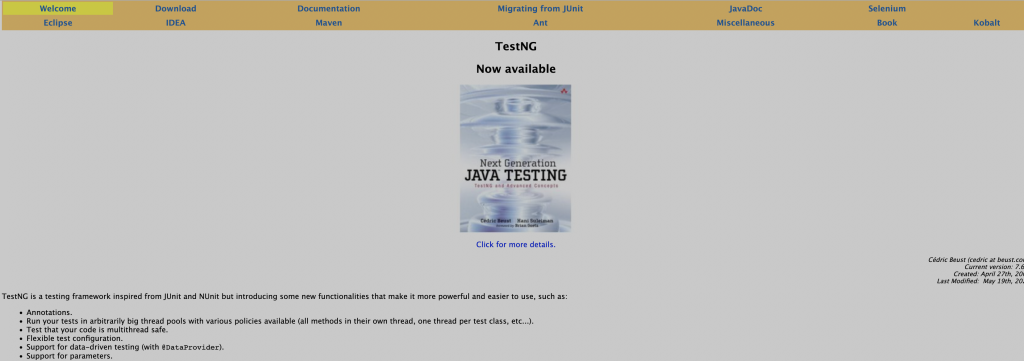
Implementing TestNG DataProviders in Real-World Scenarios
Alright, we’ve chatted about the theory, the syntax, and the nuances of the @DataProvider
annotation. But as they say, the real fun begins when you roll up your sleeves and get your hands dirty with some actual coding. Let’s dive into the practical side of things and see how DataProviders can be seamlessly integrated into your TestNG tests.
Step-by-Step: Crafting Your First DataProvider Test
- Setting the Stage: Start by importing the necessary TestNG annotations.
import org.testng.annotations.DataProvider; import org.testng.annotations.Test;
- Creating the DataProvider Method: This is where you define the data sets you want to test.
@DataProvider(name = "loginCredentials") public Object[][] provideLoginData() { return new Object[][] { {"Alice", "password123"}, {"Bob", "passBob"} }; }
- Writing the Test Method: Now, let’s write the test that will use the data from our DataProvider.
@Test(dataProvider = "loginCredentials") public void loginTest(String username, String password) { System.out.println("Logging in with: " + username + " and " + password); // Your actual login test logic here }
- Running the Test: Execute your test using your preferred IDE or through the command line. Watch as TestNG magically runs the
loginTest
method for each set of credentials you’ve provided.
Deciphering the Output
After running the test, you’ll notice the console spitting out lines for each data set. For our example, you’d see:
Logging in with: Alice and password123
Logging in with: Bob and passBob
What’s happening here? TestNG is running the loginTest
method for each pair of username and password we provided in our dataprovider. It’s like having multiple rehearsals with different songs from the setlist.
- The Takeaway: DataProviders offer a dynamic way to run tests with multiple data sets, ensuring comprehensive coverage. The output gives a clear picture of how each data set performs, allowing for targeted debugging and optimization.
In conclusion, DataProviders in TestNG are a powerful tool for data-driven testing. They allow for flexibility, reduce redundancy, and ensure that tests are comprehensive. By understanding the syntax, practical implementation, and output, testers can harness the full potential of DataProviders to enhance their testing processes.
Leveling Up with DataProviders: The Art of Inheritance in TestNG
In the world of object-oriented programming, inheritance is a game-changer. It allows for reusability, organization, and a clear structure. Now, imagine combining this power with the dynamism of TestNG DataProviders. The result? A streamlined testing process that’s both efficient and organized. Let’s delve deeper into the advanced realm of inheriting DataProviders across classes in TestNG.
- Inheriting DataProviders: Breaking Down the Walls Between Classes
Inheritance isn’t just about passing down family heirlooms; in TestNG, it’s about sharing valuable test data across different classes. By inheriting DataProviders, you can define your data sets in one class and use them in another. It’s like having a universal remote that works on multiple devices. Consider you have a base class where your DataProvider resides:
public class BaseDataProviderClass {
@DataProvider(name = "sharedData")
public Object[][] provideSharedData() {
return new Object[][] { {"Data1"}, {"Data2"} };
}
}
Now, in a separate test class, you can inherit this DataProvider and use it seamlessly:
public class TestClass extends BaseDataProviderClass {
@Test(dataProvider = "sharedData")
public void inheritedTest(String data) {
System.out.println("Testing with: " + data);
}
}
Running the inheritedTest
method will use the data from the provideSharedData
method in the base class, showcasing the power of inheritance in TestNG.
- The Perks of Decoupling DataProviders from Test Methods
- Organized Codebase: By separating DataProvider methods from test methods, you create a clear distinction between data and logic. It’s like having a dedicated pantry for ingredients and a separate kitchen for cooking.
- Reusability: One DataProvider can serve multiple test classes. Think of it as a communal garden where multiple households can pick fresh produce.
- Maintainability: Changes to the data don’t affect the test logic and vice versa. It’s akin to updating the playlist without changing the music player.
In the grand scheme of things, the advanced usage of DataProviders, especially with inheritance, amplifies the capabilities of TestNG. It not only streamlines the testing process but also ensures a clean and maintainable codebase. So, the next time you’re working with TestNG DataProviders, remember the power of inheritance and the benefits it brings to the table.
Handling Multiple Parameters in TestNG DataProviders
In the circus of software testing, handling multiple parameters can sometimes feel like juggling flaming torches while riding a unicycle. But fear not! With TestNG DataProviders, you can master this act with grace and precision. Let’s dive into the world of multi-parameter testing and see how DataProviders can be your safety net.
- The Magic of Multi-Parameter Testing with TestNG DataProviders
Testing with multiple parameters is like cooking a complex dish. Each ingredient (parameter) plays a crucial role, and missing one can change the outcome. Thankfully, with TestNG DataProviders, adding multiple ingredients to your test recipe becomes a cakewalk. Consider a scenario where you’re testing a registration form that requires a username, password, and email. Instead of running separate tests for each combination, you can use a single DataProvider to feed all the required data.
@DataProvider(name = "registrationData")
public Object[][] provideRegistrationData() {
return new Object[][] {
{"Alice", "passAlice", "alice@email.com"},
{"Bob", "passBob", "bob@email.com"}
};
}
- Crafting Tests with Multiple Parameters: A Practical Approach
Once you’ve set up your DataProvider with multiple parameters, the next step is to craft a test that can consume this data. Here’s how you can do it:
@Test(dataProvider = "registrationData")
public void registrationTest(String username, String password, String email) {
System.out.println("Registering user: " + username);
System.out.println("With email: " + email);
// Your actual registration test logic here
}
Running this test will execute the registrationTest
method for each set of data provided by our dataprovider. It’s like having a conveyor belt that delivers different sets of ingredients for each dish you’re preparing.
- Decoding the Multi-Parameter Test Output
After executing the test, your console will be bustling with activity. For our registration example, the output would look something like:
Registering user: Alice
With email: alice@email.com
Registering user: Bob
With email: bob@email.com
This output gives a clear indication of how each set of parameters was used in the test. It’s like having a play-by-play commentary for a sports match, helping you understand each move in detail.
Handling multiple parameters can seem daunting. But with the power of TestNG DataProviders, this task becomes streamlined and efficient. So, the next time you find yourself juggling multiple test parameters, remember that DataProviders are here to ensure you never drop the ball.
Integrating Method Parameters with TestNG DataProviders
In the dynamic world of software testing, efficiency is the name of the game. And when it comes to TestNG DataProviders, there’s a nifty trick up its sleeve that can supercharge your testing process: integrating method parameters. Let’s unravel this concept and see how it can be a game-changer for your tests.
- Method Parameters in DataProviders: The What and Why
Imagine being able to customize the data your DataProvider returns based on the test method that’s about to consume it. It’s like a vending machine that dispenses a specific snack based on the button you press. By passing method names as parameters to DataProviders, you can achieve this level of customization.
@DataProvider(name = "dynamicData")
public Object[][] provideData(Method m) {
if (m.getName().equals("testMethodA")) {
return new Object[][] { {"DataForA"} };
} else {
return new Object[][] { {"DataForB"} };
}
}
- Showcasing the Power of Method Parameters in Action
With our DataProvider set up to return data based on the method name, let’s see it in action:
@Test(dataProvider = "dynamicData")
public void testMethodA(String data) {
// Test logic for method A
}
@Test(dataProvider = "dynamicData")
public void testMethodB(String data) {
// Test logic for method B
}
When testMethodA
runs, it gets “DataForA” from our dataprovider, while testMethodB
gets a different set of data.
- Reaping the Rewards: Why This Approach Rocks
- Tailored Testing: Each test method gets data that’s specifically tailored for it.
- Reduced Redundancy: No need for multiple DataProviders for different test methods. One dynamic DataProvider does the job.
- Streamlined Codebase: Less code to manage and easier maintenance.
Wrapping Up
As we journey through the intricate lanes of software testing, tools like TestNG DataProviders emerge as invaluable allies. They not only offer the flexibility to test with varied data sets but also ensure comprehensive test coverage. Whether you’re a newbie or a seasoned tester, diving deep into the world of DataProviders and TestNG Parameters can significantly elevate your testing game. So, gear up, practice, and harness the full potential of these tools to make your tests more robust and efficient. Happy testing!