Java and MySQL are a popular combination for building powerful and scalable applications. Java provides a robust programming language and runtime environment that can be used to build applications of all sizes and complexities. MySQL, on the other hand, provides a fast and reliable relational database management system that is widely used across a variety of industries.
Using Java and MySQL together provides developers with a powerful toolset for building database-driven applications. Java provides a powerful JDBC API for interacting with MySQL databases, and the extensive Java class library provides a wide range of tools and utilities for building complex applications. Additionally, Java frameworks like Spring and Hibernate provide powerful tools for building web applications with MySQL backends.
Table of Contents
Setting Up Your Environment
Before diving into java crud operation with mysql, it’s essential to set up your development environment correctly. This setup involves a few critical components:
Prerequisites for Java and MySQL Integration
- JDK Installation: Ensure you have the Java Development Kit (JDK) installed on your system. The JDK is necessary for developing and running Java applications. You can download it from the official Oracle website.
- MySQL Server Setup: You’ll need a MySQL server running to store and manage your database. Download and install MySQL Community Server from the official MySQL website. Follow the installation guide to set it up on your local machine.
- JDBC Driver for MySQL: The JDBC Driver enables Java applications to interact with the MySQL database. Download the MySQL Connector/J from the MySQL website and include it in your project’s classpath.
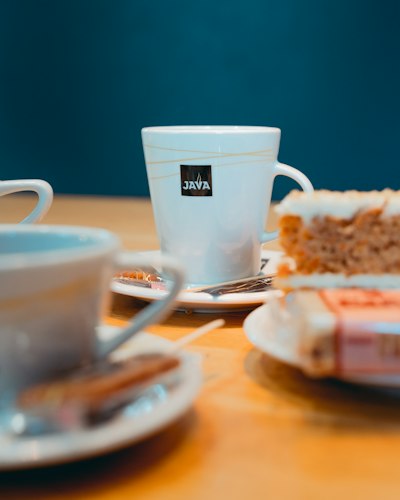
Creating a MySQL Database for CRUD Operations
To perform CRUD operations, you first need a database and a table. Here’s how you can create a simple Users
table in MySQL:
Step-by-Step Guide to Creating a Sample Database
Execute the following SQL script in your MySQL Workbench or command line client to create a database named SampleDB
and a Users
table:
CREATE DATABASE SampleDB;
USE SampleDB;
CREATE TABLE `users` (
`user_id` int(11) NOT NULL AUTO_INCREMENT,
`username` varchar(45) NOT NULL,
`password` varchar(45) NOT NULL,
`fullname` varchar(45) NOT NULL,
`email` varchar(45) NOT NULL,
PRIMARY KEY (`user_id`)
);
This script sets up a basic structure for storing user information, including a unique ID, username, password, full name, and email address.
Establishing a Connection with MySQL
To interact with the MySQL database from Java, you need to establish a connection using JDBC.
Understanding JDBC Interfaces and Classes
JDBC provides several interfaces and classes for database operations:
- DriverManager: This class manages a list of database drivers. It is used to establish a connection to the database.
- Connection: Represents a connection (session) with a specific database. SQL statements are executed and results are returned within the context of a connection.
- Statement & PreparedStatement: Interfaces for executing SQL statements.
PreparedStatement
allows you to execute parameterized queries. - ResultSet: Represents the result set of a database query.
- SQLException: An exception that provides information on a database access error or other errors.
Code Snippet to Connect to MySQL Database
Here’s a basic example of how to connect to the SampleDB
database using JDBC:
String dbURL = "jdbc:mysql://localhost:3306/SampleDB";
String username = "root";
String password = "yourpassword";
try (Connection conn = DriverManager.getConnection(dbURL, username, password)) {
System.out.println("Connected to the database successfully.");
} catch (SQLException e) {
e.printStackTrace();
}
This code snippet demonstrates the use of the try-with-resources statement to automatically close the database connection, simplifying resource management.
Performing CRUD Operations
Executing CRUD operations is a fundamental aspect of interacting with databases in Java applications. This section delves into how to insert data into MySQL using JDBC, a crucial part of managing dynamic content in your applications.
Inserting Data into MySQL Using JDBC
Inserting data into a MySQL database from a Java application involves two main steps: preparing the SQL insert statement and executing it through JDBC. This process is streamlined by using the PreparedStatement
interface, which helps in setting dynamic values in the SQL query securely.
- Preparing the INSERT Statement: The first step is to write the SQL query for inserting data. Unlike a regular
Statement
, aPreparedStatement
allows for setting parameters dynamically, which helps prevent SQL injection attacks.
String insertQuery = "INSERT INTO users (username, password, fullname, email) VALUES (?, ?, ?, ?)";
- Executing the INSERT Statement: Once the query is prepared, the next step is to set the values for each parameter using the appropriate
set
methods provided by thePreparedStatement
. After setting the values, the statement is executed to insert the data into the database.
try (Connection conn = DriverManager.getConnection(dbURL, username, password);
PreparedStatement pstmt = conn.prepareStatement(insertQuery)) {
pstmt.setString(1, "john_doe");
pstmt.setString(2, "securepassword");
pstmt.setString(3, "John Doe");
pstmt.setString(4, "john.doe@example.com");
int rowsAffected = pstmt.executeUpdate();
if (rowsAffected > 0) {
System.out.println("A new user was inserted successfully!");
}
} catch (SQLException e) {
e.printStackTrace();
}
In this example, the try-with-resources
statement is used to automatically close the Connection
and PreparedStatement
objects, thus managing resources efficiently. The setString
method assigns values to the placeholders (?
) in the SQL query based on their respective positions. The executeUpdate
method executes the SQL statement, which in this case, inserts a new record into the users
table. The method returns the number of rows affected, which can be used to verify that the insertion was successful.
This approach to inserting data into MySQL using JDBC ensures that your Java application can dynamically and securely manage database records.
Retrieving Data from MySQL
Retrieving data is a core component of CRUD operations, allowing applications to display and utilize stored information. In Java, this is achieved through crafting SELECT queries and navigating the ResultSet
.
- Crafting SELECT Queries to Fetch Data: To retrieve data, you formulate a SELECT SQL statement. This statement can be executed using a
Statement
orPreparedStatement
object for added security and flexibility.
String selectQuery = "SELECT * FROM users";
- Navigating the ResultSet: After executing the query, a
ResultSet
object is returned, which is iterated to access the retrieved data.
try (Connection conn = DriverManager.getConnection(dbURL, username, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(selectQuery)) {
while (rs.next()) {
System.out.println("Username: " + rs.getString("username") + ", Email: " + rs.getString("email"));
}
} catch (SQLException e) {
e.printStackTrace();
}
Updating Data in MySQL
Updating existing records is essential for maintaining accurate and current data within your database.
- Formulating and Executing UPDATE Statements: Similar to inserting data, updating data in MySQL involves preparing an UPDATE SQL statement and executing it.
String updateQuery = "UPDATE users SET email = ? WHERE username = ?";
- Parameter Handling in PreparedStatement: Parameters in the UPDATE statement are set using the
PreparedStatement
to ensure data integrity.
try (Connection conn = DriverManager.getConnection(dbURL, username, password);
PreparedStatement pstmt = conn.prepareStatement(updateQuery)) {
pstmt.setString(1, "new.email@example.com");
pstmt.setString(2, "john_doe");
pstmt.executeUpdate();
System.out.println("User data updated successfully.");
} catch (SQLException e) {
e.printStackTrace();
}
Deleting Data from MySQL
Removing data from a database is a critical operation that must be handled with care to avoid unintended data loss.
- Constructing and Executing DELETE Statements: To delete records, you prepare a DELETE SQL statement.
String deleteQuery = "DELETE FROM users WHERE username = ?";
- Using PreparedStatement to Set Query Parameters: The
PreparedStatement
is used to securely set the parameters for the DELETE operation.
try (Connection conn = DriverManager.getConnection(dbURL, username, password);
PreparedStatement pstmt = conn.prepareStatement(deleteQuery)) {
pstmt.setString(1, "john_doe");
int rowsAffected = pstmt.executeUpdate();
if (rowsAffected > 0) {
System.out.println("User deleted successfully.");
}
} catch (SQLException e) {
e.printStackTrace();
}
Best Practices for JDBC Programming
When working with JDBC, adhering to best practices ensures efficient and secure database interactions.
- Error Handling and Resource Management: Proper error handling and resource management are crucial. The try-with-resources statement is highly recommended for managing database connections, as it ensures that all resources are automatically closed at the end of the block.
try (Connection conn = DriverManager.getConnection(dbURL, username, password)) {
// Perform database operations
} catch (SQLException e) {
// Handle exceptions
}
This approach minimizes the risk of resource leaks and ensures that your Java application interacts with MySQL databases efficiently and safely.
FAQs
What is JDBC in Java?
JDBC stands for Java Database Connectivity, a standard Java API for database-independent connectivity between the Java programming language and a wide range of databases. It provides a unified interface for accessing and manipulating databases from Java applications.
How can I handle SQL exceptions in JDBC?
SQL exceptions in JDBC are managed through try-catch blocks. When an SQL exception occurs, it’s caught by the catch block, allowing the developer to handle it appropriately, whether by logging the error, rolling back transactions, or notifying the user.