Laravel, a popular PHP framework, boasts a range of features designed to simplify web application development. Eloquent provides an intuitive way to interact with databases, and among its many capabilities is the concept of “soft deletes.” This feature allows developers to remove records from a database without permanently deleting them, offering a layer of data protection and flexibility.
Table of Contents
What is Soft Delete?
In database management, when a record is deleted, it’s typically removed permanently. This is known as a hard delete. However, there are scenarios where a record needs to be hidden or rendered inactive without being permanently removed. This is where soft deletes come into play. Instead of erasing the record, a timestamp is added to a specific column (usually deleted_at
). This indicates that the record has been soft deleted, even though it remains in the database.
$user->delete(); // This will soft delete the record
Why Use Soft Deletes?
Opting for soft deletes over hard deletes offers several advantages. Firstly, it provides a safety net against accidental deletions. If a record is mistakenly removed, it can be easily restored. Secondly, for auditing purposes, having a trail of all records, including those that have been “deleted,” can be invaluable. Lastly, in terms of user experience, soft deletes can allow for features like “undo” actions or temporary archive states, enhancing the overall user interaction with the application. In essence, using Laravel soft delete functionality ensures data integrity while offering more flexibility in data management.
Implementing Soft Deletes in Laravel
Setting Up the Migration
To leverage the Laravel soft delete functionality, the first step involves modifying the database schema. Specifically, you need to add a deleted_at
column to the table, which will store the timestamp when a record is soft deleted. Laravel makes this process straightforward with the softDeletes
method.
Schema::table('users', function (Blueprint $table) {
$table->softDeletes();
});
This code snippet will add the necessary deleted_at
column to the ‘users’ table.
Using the SoftDeletes Trait
Once the migration is set up, the next step is to inform the Eloquent model about the soft delete capability. This is achieved by using the SoftDeletes
trait in the model.
use Illuminate\Database\Eloquent\SoftDeletes;
class User extends Model {
use SoftDeletes;
}
With this trait in place, the model is now equipped to handle soft delete operations seamlessly.
Performing Soft Delete Operations
Soft deleting a record is as simple as calling the delete
method on an Eloquent model instance.
$user = User::find(1);
$user->delete(); // This will soft delete the user with ID 1
To retrieve soft-deleted records, Laravel provides the withTrashed
method.
$users = User::withTrashed()->get();
This will fetch all users, including those that have been soft deleted.
Restoring Soft Deleted Records
The Restore Method
There might be situations where a soft-deleted record needs to be brought back to its active state. Laravel provides the restore
method for this purpose.
$user = User::withTrashed()->find(1);
$user->restore(); // This will restore the soft-deleted user with ID 1
Bulk Restoring Records
In cases where multiple records need to be restored simultaneously, Laravel offers a convenient way to achieve this.
User::withTrashed()->where('account_type', 'premium')->restore();
The above code will restore all premium users that have been soft deleted.
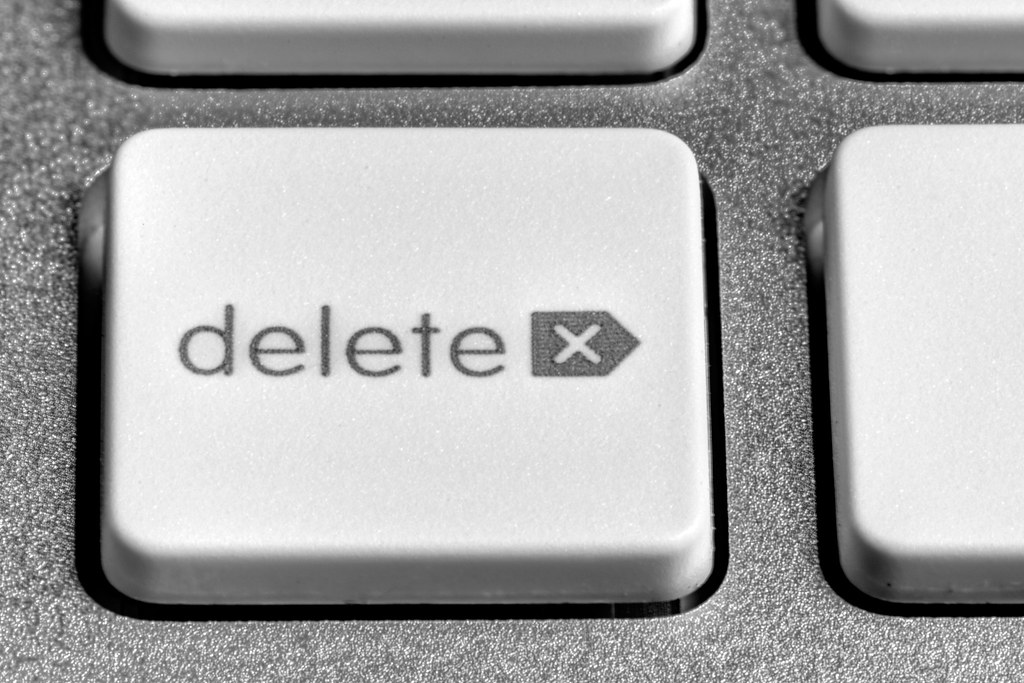
Permanently Deleting Records
The Force/Hard Delete Method
While the Laravel soft delete feature is invaluable for preserving data, there are instances when a record needs to be removed permanently. For such cases, Laravel offers the forceDelete
method. When applied to a model that has been soft deleted, this method will permanently remove the record from the database.
$user = User::withTrashed()->find(1);
$user->forceDelete(); // This will permanently delete the user with ID 1
Bulk Force/Hard Deleting
To permanently delete multiple soft-deleted records in one go, you can combine query builder methods with forceDelete
.
User::onlyTrashed()->where('account_status', 'inactive')->forceDelete();
This command will permanently remove all inactive users that have been soft deleted.
Querying Soft Deleted Records
Using the withTrashed
Method
Laravel provides a seamless way to retrieve both active and soft-deleted records using the withTrashed
method. This is particularly useful when you want a comprehensive view of all records, regardless of their deletion status.
$allUsers = User::withTrashed()->get();
This query will fetch all users, including those that have undergone the Laravel soft delete process.
Using the onlyTrashed
Method
If you’re interested in querying only the soft-deleted records, Laravel’s onlyTrashed
method comes in handy.
$deletedUsers = User::onlyTrashed()->get();
This will retrieve exclusively the users that have been soft deleted, allowing for targeted operations or audits on these specific records.
FAQs
How does Laravel differentiate between soft deleted and non-deleted records?
Laravel uses the deleted_at
timestamp column to differentiate. If a record has a null value in this column, it’s considered active. However, if there’s a timestamp, it indicates the record has been soft deleted.
Can I customize the name of the deleted_at
column?
Yes, you can. While deleted_at
is the default, you can specify a different column name in your Eloquent model by defining a DELETED_AT
constant with your desired column name.
How can I disable soft deletes for specific queries?
You can utilize the withoutTrashed
method. This ensures that your query results exclude soft-deleted records, giving you only active data.
Is it possible to apply global scopes to soft deleted records?
Absolutely. Laravel allows you to apply global scopes to your queries, and this includes those that involve soft-deleted records. This way, you can define common query constraints for soft deletes.
Soft deletes in Laravel offer a robust way to manage data deletions without permanent loss. By understanding and implementing this feature correctly, developers can ensure data integrity and enhance application functionality.