In the vast realm of the Java ecosystem, testing frameworks stand as the unsung heroes, ensuring that our code not only works but thrives. They’re the guardians, the watchers on the walls of our applications. And when it comes to these guardians, two names often echo louder than the rest: JUnit and TestNG. But how do they stack up against each other? Here is a sneak peek…
Feature | JUnit | TestNG |
---|---|---|
Basic Testing | Supported | Supported |
Annotations | @Test , @Before , @After , etc. | @Test , @BeforeMethod , etc. |
Parameterized Testing | @ParameterizedTest with sources | @DataProvider and @Parameters |
Grouping of Tests | Limited, mainly via suites | @Test(groups="...") with XML |
Dependent Tests | Not Supported | @Test(dependsOnMethods="...") |
Parallel Execution | Limited support | Built-in with XML configurations |
Custom Test Naming | @DisplayName | Not directly supported |
Timeouts | @Test(timeout=...) | @Test(timeOut=...) |
Exception Testing | @Test(expected=...) | @Test(expectedExceptions=...) |
Test Suites | @Suite | XML-based suites |
Ignore Tests | @Ignore | @Test(enabled=false) |
Order of Execution | @FixMethodOrder | priority attribute |
Setup & Teardown | @BeforeEach , @BeforeAll , etc. | @BeforeMethod , @BeforeClass , etc. |
Community & Ecosystem | Larger, more mature | Growing, more advanced features |
Table of Contents
Diving Deep into Test Setup: TestNG vs JUnit
When setting up tests, both JUnit and TestNG have their own flair and style. Let’s explore how they do it.
JUnit Test Setup
JUnit, being one of the pioneers in the Java testing world, has a set of annotations that help in initializing and tearing down tests. These are:
@BeforeEach
: Prepares the stage before each test.@AfterEach
: Cleans up after each performance.@BeforeAll
: Sets the scene before the entire test suite.@AfterAll
: Bids adieu once all tests are done.
Here’s a simple Java class showcasing these annotations:
import org.junit.jupiter.api.*;
public class JUnitExampleTest {
@BeforeAll
static void setupOnce() {
System.out.println("Setting up the environment...");
}
@BeforeEach
void setup() {
System.out.println("Setting up before each test...");
}
@Test
void sampleTest() {
System.out.println("Running the sample test...");
}
@AfterEach
void tearDown() {
System.out.println("Cleaning up after the test...");
}
@AfterAll
static void tearDownOnce() {
System.out.println("Cleaning up post all tests...");
}
}
TestNG Test Setup
On the other side of the spectrum, TestNG brings its own set of annotations for test setup and teardown. These include:
@BeforeMethod
: A prelude to each test method.@AfterMethod
: The curtain call post each test.@BeforeClass
: The overture before the class tests.@AfterClass
: The final bow after all class tests.
Here’s how you’d use these annotations in a Java class:
import org.testng.annotations.*;
public class TestNGExampleTest {
@BeforeClass
void initClass() {
System.out.println("Initializing the test class...");
}
@BeforeMethod
void setup() {
System.out.println("Pre-test setup...");
}
@Test
void exampleTest() {
System.out.println("Executing the example test...");
}
@AfterMethod
void clean() {
System.out.println("Post-test cleanup...");
}
@AfterClass
void finishClass() {
System.out.println("Wrapping up the test class...");
}
}
In the battle of how to integrate TestNG vs JUnit, understanding their test setup methodologies is the first step. As we delve deeper, the distinctions and similarities become even more fascinating.
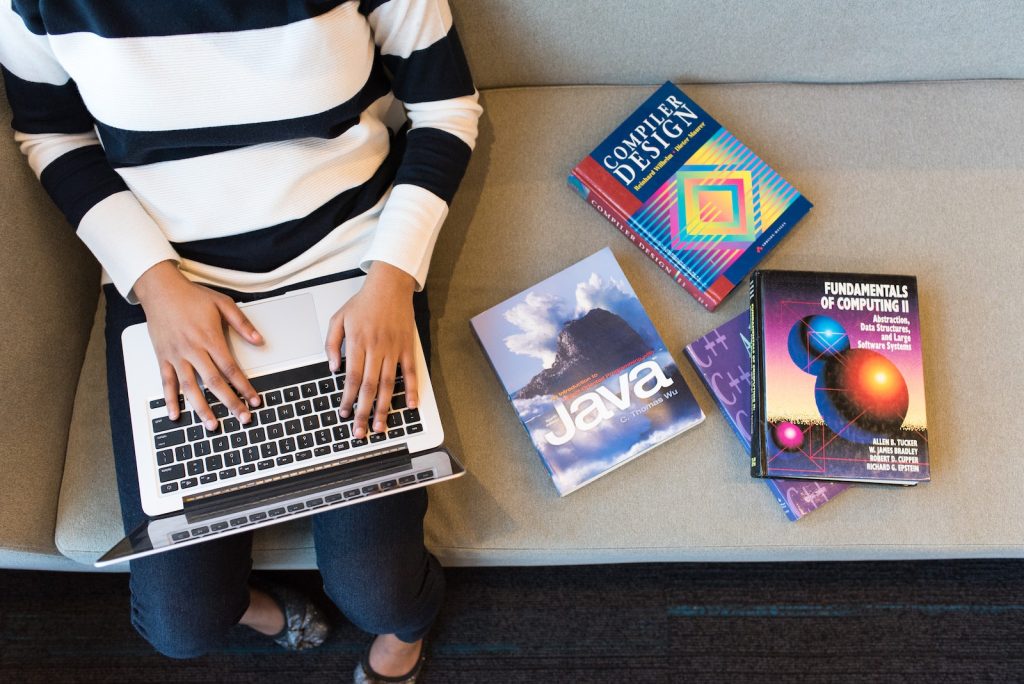
Ignoring Tests
In the world of testing, not all tests are born equal. Sometimes, we need to sideline a few for various reasons. Both JUnit and TestNG offer ways to do this, but their methods differ.
JUnit: Meet the @Ignore
annotation. It’s like the “do not disturb” sign you hang on hotel room doors. Slap this on a test, and JUnit will walk right past it.
import org.junit.*;
public class JUnitSelectiveTest {
@Ignore
@Test
public void ignoredTest() {
// This test will not run
}
}
TestNG: TestNG takes a more parameterized route. Using the @Test
annotation itself, you can set the “enabled” parameter to false. It’s like telling TestNG, “Not this one, please!”
import org.testng.annotations.Test;
public class TestNGSelectiveTest {
@Test(enabled = false)
public void skippedTest() {
// This test will be skipped
}
}
Grouping and Running Tests Collections
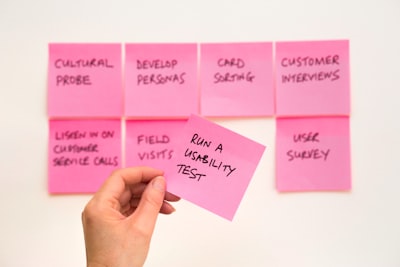
One of the things you’ll notice is their distinct ways of running tests collectively.
JUnit:
@Suite
: Think of this as the master playlist of your tests.@SelectPackages
: A way to pick entire packages for testing.@SelectClasses
: Handpick specific classes to be part of the test run.
import org.junit.platform.suite.api.SelectPackages;
import org.junit.platform.suite.api.Suite;
@Suite
@SelectPackages("com.example.tests")
public class JUnitGroupTest {}
TestNG: TestNG uses XML configurations to group tests. But, it also has the @Test(groups=”groupName”)
annotation, allowing you to categorize tests and run them based on these categories.
import org.testng.annotations.Test;
public class TestNGGroupTest {
@Test(groups = "integration")
public void integrationTest1() {
// This is an integration test
}
}
Exception Testing
Errors happen. And when they do, it’s essential to know how our testing frameworks react. Both JUnit and TestNG have mechanisms to test exceptions, ensuring that our code behaves as expected, even when things go awry.
JUnit’s Exception Handling: JUnit uses the expected
parameter within the @Test
annotation. It’s like setting a trap and waiting for the specific exception to spring it.
import org.junit.*;
public class JUnitExceptionTest {
@Test(expected = ArithmeticException.class)
public void testDivisionByZero() {
int result = 10 / 0;
}
}
TestNG Exception Handling: TestNG, not wanting to be left behind, uses a similar approach. The @Test
annotation has an expectedExceptions
parameter to catch those pesky exceptions.
import org.testng.annotations.Test;
public class TestNGExceptionTest {
@Test(expectedExceptions = ArithmeticException.class)
public void divideByZeroTest() {
int outcome = 10 / 0;
}
}
In the grand scheme of things, understanding these nuances can make the journey of integrating TestNG vs JUnit smoother and more informed.
Parameterized Tests: JUnit 5 vs TestNG
When it comes to feeding a variety of data into our tests, parameterized tests are the unsung heroes. They allow us to run the same test logic with different input values, ensuring our code is robust and versatile. Both JUnit 5 and TestNG offer this feature, but their approaches are as distinct as their personalities.
JUnit 5’s Data Sources:
@ValueSource
: Simple values, like a string or a number, can be fed directly.@EnumSource
: Enumerations, anyone? This is your go-to.@MethodSource
: For when you need a method to provide your test data.@CsvSource
: CSV values can be a source too!
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
public class JUnitParameterizedTest {
@ParameterizedTest
@ValueSource(strings = {"apple", "banana", "cherry"})
void testFruits(String fruit) {
assertNotNull(fruit);
}
}
TestNG’s Data Sources:
@Parameter
: Directly feed parameters from your XML configuration.@DataProvider
: A method that returns data in a structured manner.
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class TestNGParameterizedTest {
@DataProvider(name = "fruitProvider")
public Object[][] createData() {
return new Object[][] {{"apple"}, {"banana"}, {"cherry"}};
}
@Test(dataProvider = "fruitProvider")
public void testFruits(String fruit) {
assertNotNull(fruit);
}
}
Test Timeouts
In the world of testing, time is of the essence. We don’t want tests that run indefinitely, hogging resources. Both JUnit and TestNG recognize this and provide mechanisms to ensure our tests are time-efficient.
JUnit: Simply use the timeout
parameter in the @Test
annotation. If the test exceeds this time, it’s considered a failure.
import org.junit.Test;
public class JUnitTimeoutTest {
@Test(timeout = 1000) // 1 second
public void timeSensitiveTest() {
// Some test logic here
}
}
TestNG: TestNG uses the timeOut
attribute in its @Test
annotation. If the clock runs out before the test completes, it’s game over.
import org.testng.annotations.Test;
public class TestNGTimeoutTest {
@Test(timeOut = 1000) // 1 second
public void testWithTimeLimit() {
// Test logic that shouldn't take too long
}
}
When pondering how to integrate TestNG vs JUnit, understanding these timeout mechanisms can be a game-changer, ensuring your tests are not just accurate but also swift.
Dependent Tests
In the vast landscape of testing, there’s a feature that makes TestNG stand out from the crowd: dependent tests. Imagine a scenario where one test’s outcome influences whether another test runs. Sounds intriguing, right? That’s precisely what TestNG brings to the table with its dependsOnMethods
attribute.
TestNG’s Dependent Tests: By using the dependsOnMethods
attribute within the @Test
annotation, you can specify that a particular test method should only run if another test method passes.
import org.testng.annotations.Test;
public class TestNGDependentTests {
@Test
public void loginTest() {
System.out.println("Logging in...");
// Logic for login test
}
@Test(dependsOnMethods = {"loginTest"})
public void dashboardTest() {
System.out.println("Accessing dashboard...");
// Logic for dashboard test after successful login
}
}
In the above example, dashboardTest
will only execute if loginTest
passes. It’s a neat way to ensure the sequence and dependency of tests, especially when you’re figuring out how to integrate TestNG vs JUnit.
Order of Test Execution
The sequence in which tests run can be crucial. Both JUnit and TestNG offer ways to control this order, ensuring tests run in the desired sequence.
JUnit Test Order: JUnit provides the @FixMethodOrder
annotation, which allows you to dictate the order of test execution.
import org.junit.FixMethodOrder;
import org.junit.Test;
import org.junit.runners.MethodSorters;
@FixMethodOrder(MethodSorters.NAME_ASCENDING)
public class JUnitOrderTest {
@Test
public void A_firstTest() {
// This test will run first
}
@Test
public void B_secondTest() {
// This test will run second
}
}
TestNG’s Test Order: TestNG uses the priority
attribute and the already mentioned dependsOnMethods
to control test order.
import org.testng.annotations.Test;
public class TestNGOrderTest {
@Test(priority = 1)
public void initiate() {
// This test will run first
}
@Test(priority = 2, dependsOnMethods = {"initiate"})
public void proceed() {
// This test will run second, after 'initiate'
}
}
Understanding the order of test execution is pivotal, especially when integrating TestNG vs JUnit. It ensures a logical flow and can prevent potential pitfalls in the testing process.
Custom Test Naming
When you’re knee-deep in test results, deciphering what each test does can feel like translating an ancient script. Custom test naming is the Rosetta Stone of this world, making results more readable and understandable.
JUnit 5’s Naming: JUnit 5 introduces the @DisplayName
annotation, allowing you to give your tests descriptive names that are human-friendly.
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Test;
public class JUnitNamingTest {
@Test
@DisplayName("When user logs in with valid credentials")
public void successfulLoginTest() {
// Test logic here
}
}
TestNG’s Naming: Currently, TestNG doesn’t have a direct counterpart to JUnit’s @DisplayName
. It’s one of the areas where TestNG aficionados are eagerly awaiting enhancements.
Conclusion: TestNG vs JUnit
As we wrap up our deep dive into the world of TestNG vs JUnit, it’s evident that both have their strengths and quirks. From test setups to exception handling, from parameterized tests to order of execution, we’ve seen how each framework shines and where they differ.
If you’re looking for flexibility and advanced features, TestNG might be your go-to. However, if you value simplicity and a vast community, JUnit could be the better choice. Ultimately, when pondering to use TestNG vs JUnit, consider your project’s specific needs.
FAQs
Why is TestNG considered better than JUnit in some scenarios?
TestNG offers features like dependent tests and enhanced grouping, making it a favorite for complex testing scenarios.
How does the user community for JUnit compare to TestNG?
JUnit, being older, has a larger community. This means more resources, tutorials, and third-party integrations. TestNG, while younger, has a passionate community and is growing steadily.
Are there any additional features in TestNG compared to JUnit?
Yes, TestNG provides unique features like dependent tests and XML-based test configurations, which aren’t native to JUn